10 Essential Cybersecurity Practices Every Developer Should Know
By Adam Hultman
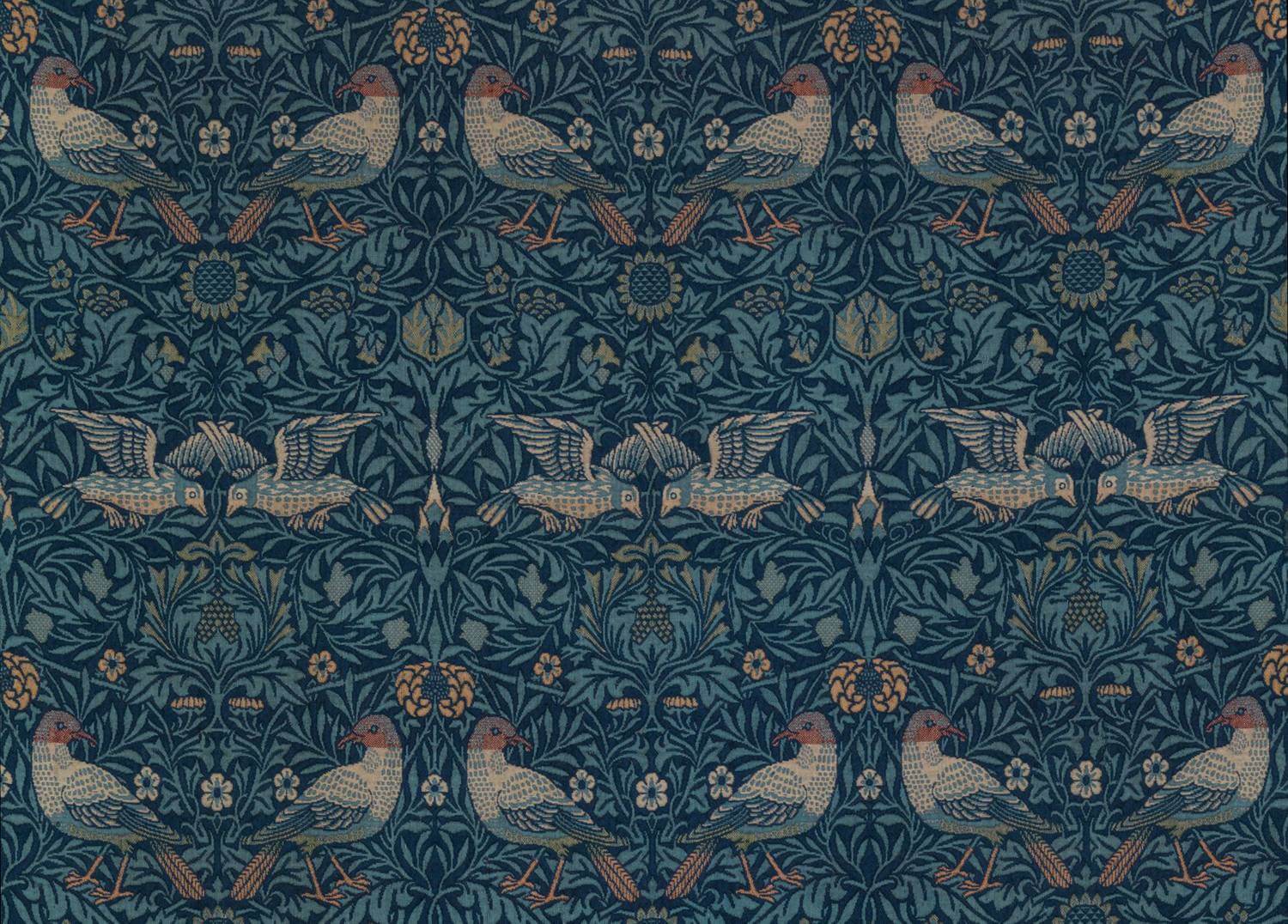
In the world of software development, cybersecurity is no longer just a nice-to-have; it’s a necessity. Whether you’re building a small personal project or deploying a production app, integrating basic security practices into your workflow can save you—and your users—a lot of trouble. In this post, I’ll walk you through ten essential cybersecurity practices that every developer should know, with a focus on practical, easy-to-implement tips.
1. Secure Your APIs Like a Vault
APIs are the backbone of modern applications, but they’re also a common target for attackers. Securing your APIs means controlling who can access them and how they’re accessed. The basics include using HTTPS for secure data transfer and ensuring that API keys or tokens are kept safe—never hardcode them in your source files.
If you’re using a service like AWS or Firebase, take advantage of API gateways and throttling features to limit the number of requests per user. And always validate input, even for endpoints that seem harmless. A poorly validated query parameter can lead to security nightmares like SQL injection (more on that below).
2. Authentication and Authorization: Know the Difference
Authentication and authorization are often used interchangeably, but they serve very different purposes. Authentication is about verifying who the user is, while authorization is about controlling what they can access once they’re authenticated.
For implementing authentication, OAuth 2.0 is the standard choice. It’s robust, widely supported, and provides a flow that’s both secure and user-friendly. If you need to verify user identity between client and server, JWT (JSON Web Tokens) is a great option. It allows you to issue tokens that can be stored on the client side and checked by the server for each request.
Here’s a quick example of generating a JWT:
1
2
3
4
5
6
7
8
const jwt = require('jsonwebtoken');
const token = jwt.sign(
{ userId: 123 },
process.env.JWT_SECRET,
{ expiresIn: '1h' }
);
console.log('Generated JWT:', token);
Make sure to keep your JWT secrets safe and rotate them periodically. A compromised secret can allow attackers to forge tokens and access protected resources.
3. Encrypt Sensitive Data
Encryption is your first line of defense when it comes to protecting sensitive data. If you’re storing user passwords, hash them before saving them to your database. Use libraries like bcrypt
to add a salt and hash the password:
1
2
3
4
const bcrypt = require('bcrypt');
const saltRounds = 10;
const hashedPassword = await bcrypt.hash('user-password', saltRounds);
Storing passwords in plain text is an absolute no-go, and using a basic hashing function like MD5 is no longer enough. Always choose a hashing algorithm that is designed to be slow, like bcrypt
or argon2
, which makes it harder for attackers to crack the hashes using brute force.
For data that needs to be decrypted later, such as API keys or sensitive user data, use symmetric encryption like AES (Advanced Encryption Standard). However, be careful where you store the keys—using environment variables or secure key management services like AWS KMS is a safer bet.
4. Validate All User Input
User input is one of the easiest ways for attackers to manipulate your application if it’s not properly handled. A common mistake is assuming that client-side validation is enough. It’s not. Always validate input on the server side, even if you’ve already done it on the client.
For example, if you’re accepting a user’s email address, use a regular expression or a library to ensure it’s in a valid format before processing it. This can prevent simple attacks like inputting malicious code in form fields.
1
2
3
4
function isValidEmail(email) {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(email);
}
5. Protect Against SQL Injection
SQL injection happens when attackers can manipulate your SQL queries by inserting malicious input. If you’re using traditional SQL databases, parameterized queries or prepared statements can prevent this. Most libraries and ORM frameworks like Sequelize for Node.js or SQLAlchemy for Python support parameterized queries:
1
2
3
4
const user = await db.query(
'SELECT * FROM users WHERE email = ?',
[userEmail]
);
Using a parameterized query like this prevents attackers from injecting SQL commands into your database queries. Never directly concatenate user input into SQL queries—it’s like opening the front door to your database.
6. Avoid Cross-Site Scripting (XSS)
XSS attacks occur when malicious scripts are injected into your web pages, allowing attackers to steal cookies, session tokens, or other sensitive information. To protect against XSS, you need to ensure that any data you output to the page is properly sanitized and encoded.
In a React app, the default behavior is already quite safe, but you still need to be careful with dangerouslySetInnerHTML
. If you need to render HTML from user input, use libraries like DOMPurify
to sanitize it first:
1
2
3
import DOMPurify from 'dompurify';
const safeHtml = DOMPurify.sanitize(userInputHtml);
This will strip out any potentially dangerous tags or scripts from the user’s input before rendering it on the page.
7. Use HTTPS Everywhere
If your website is accessible over HTTP, you’re putting your users’ data at risk. HTTPS ensures that the data exchanged between the server and client is encrypted, protecting against man-in-the-middle attacks.
Services like Let’s Encrypt make it easy to get an SSL certificate for free. If you’re using hosting platforms like Vercel or Netlify, they handle HTTPS for you automatically. But if you’re self-hosting, make sure to set up a redirect from HTTP to HTTPS and keep your certificates updated.
8. Regularly Perform Security Audits
Think of a security audit as a health check-up for your application. It’s not just something you do when things go wrong—it’s something that should be part of your regular development cycle.
There are automated tools like OWASP ZAP that can scan your application for common vulnerabilities. For Node.js projects, npm audit can quickly check for vulnerable packages:
1
npm audit
However, automated tools can’t catch everything. Periodically review your authentication logic, database queries, and any external services you integrate with. Security audits might feel like extra work, but they’re far easier than cleaning up after a data breach.
9. Monitor Logs for Unusual Activity
Logging is essential not just for debugging, but also for detecting security incidents. Track login attempts, failed API requests, and any changes to user accounts. A sudden spike in failed login attempts, for example, might indicate a brute-force attack.
Services like Loggly, Datadog, or even AWS’s CloudWatch can help you centralize and monitor logs. Don’t forget to rotate your logs and store them securely to avoid filling up your server or exposing sensitive information.
10. Keep Dependencies Up-to-Date
A lot of vulnerabilities come from outdated dependencies. It’s tempting to ignore the little red warnings in your terminal, but they’re there for a reason. Tools like npm
and yarn
provide commands to update packages, and services like Dependabot can automatically create pull requests to update dependencies for you:
1
npm update
Even better, consider using a service like Snyk to monitor for vulnerabilities in your dependencies. It goes beyond simple version checks, alerting you when a package has a known security issue and needs attention.
Security might seem intimidating, but it doesn’t have to be. By integrating these ten practices into your workflow, you’ll create applications that are not only functional but also secure. It’s a lot like locking your doors at night—you don’t expect a break-in, but it’s better to be prepared. And in today’s world of ever-evolving threats, a little preparation goes a long way in keeping your apps safe.